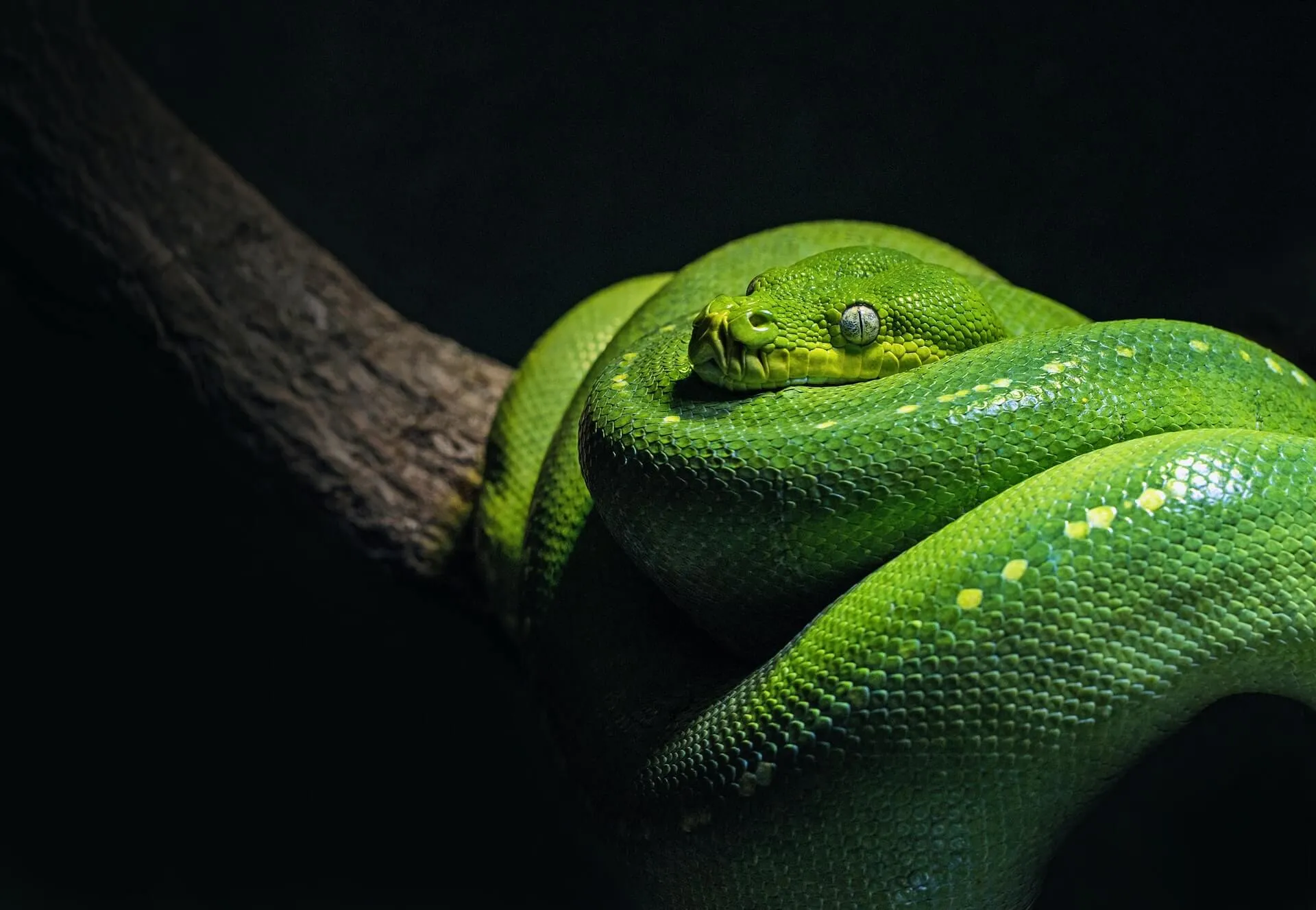
Mocking global variables in Python tests
I attempted to test global variables in Python, specifically for a script that contains global variables. After struggling to do so, I found a simple solution that I can share with you.
🏃 The quick solution
ENVS = { 'real_value': True, 'another_real_value': False }
Now, let’s go to the test file.
mocker.patch("demo_file.ENVS", new={'MOCKED_NONE': None, 'MOCKED_EMPTY': ''})
Next, the ENVS
variable will be mocked in your demo_file
.
✏️ Solution with description
Requirements
To simplify the process, I recommend using the pytest-mock
library: https://pypi.org/project/pytest-mock/
What I was trying to do?
Firstly, suppose you have a file called demo_file.py
with the following content (where the global variable is located):
import os
ENVS = { 'real_value': os.environ('REAL_VALUE'), 'another_real_value': os.environ('ANOTHER_REAL_VALUE') }
def simple_method(): print(ENVS) return 0
If you try to execute simple_method
, it will print the dictionary with real_value
and another_real_value
there.
Next, we have the following test file.
from demo_file import simple_method
def test_if_simple_method_is_working(): assert simple_method() == 0
I know the test seems simple, but it’s important to make sure that it returns a zero value after printing the values of the ENV variable.
👾 Mocking the Variable
However, as you can see, we are calling environment variables on the current computer. If you write your tests to expect those variables on your CI/CD server, the tests will fail. How can we avoid this and tell our test server (or test pipeline) that we want specific fixed values?
If you have installed the package mentioned in the requirements section, it is easy to start mocking values. We only need to add a single line.
from demo_file import simple_method
@mocker.patch("demo_file.ENVS", new={'MOCKED_REAL_VALUE': None, 'MOCKED_ANOTHER_REAL_VALUE': ''})def test_if_simple_method_is_working(): assert simple_method() == 0
Your tests will not execute the real ENVS variable and, therefore, will not call os.environ
.